Getting Started Guide
Tableau Hyper API
Overview
The Hyper API contains a set of functions you can use to automate your interactions with Tableau extract (.hyper) files. You can use the API to create new extract files or to open existing files, and then insert, delete, update, or read data from those files. Using the Hyper API developers and administrators can:
- Create extract files for data sources not currently supported by Tableau.
- Automate custom extract, transform and load (ETL) processes (for example, implement rolling window updates or custom incremental updates).
- Retrieve data from an extract file.
Pre-requisite:
Download the Hyper API library for your platform and programming language of choice. For information about getting started and installing and setting up your environment, see Install Tableau Hyper API.
- Import the Hyper API library
- Start the Hyper API process
- Open a connection to one or more Hyper files
- Create tables and insert, update, delete, and read data

After you have downloaded and installed the library, you’ll need to import or include the library in the code that you write. The name of the library will vary depending upon the programming language and client library you are using. Importing the library is simple.
Python
For example, the library is named tableauhyperapi
for the Python client library. You can import just the classes that you need from the library, or import the whole library.
from tableauhyperapi import HyperProcess, Connection, TableDefinition, SqlType, Telemetry, Inserter, CreateMode
C++
The C++ client library is called hyperapi.hpp
. The library is in the include\hyperapi
folder when you install the Hyper API library for C++. The hyperapi.hpp
file in turn includes the other classes in that folder that make up the API. For convenience and to keep your source code uncluttered, you only need to include the hyperapi.hpp
file.
#include <
hyperapi/hyperapi.hpp>
.NET (C#)
using Tableau.HyperAPI;
Java
import
com.tableau.hyperapi.*
;
Or you can import just the classes that you need from the library.
import com.tableau.hyperapi.Connection; import com.tableau.hyperapi.HyperProcess; import com.tableau.hyperapi.Result; import com.tableau.hyperapi.ResultSchema; import com.tableau.hyperapi.Telemetry;
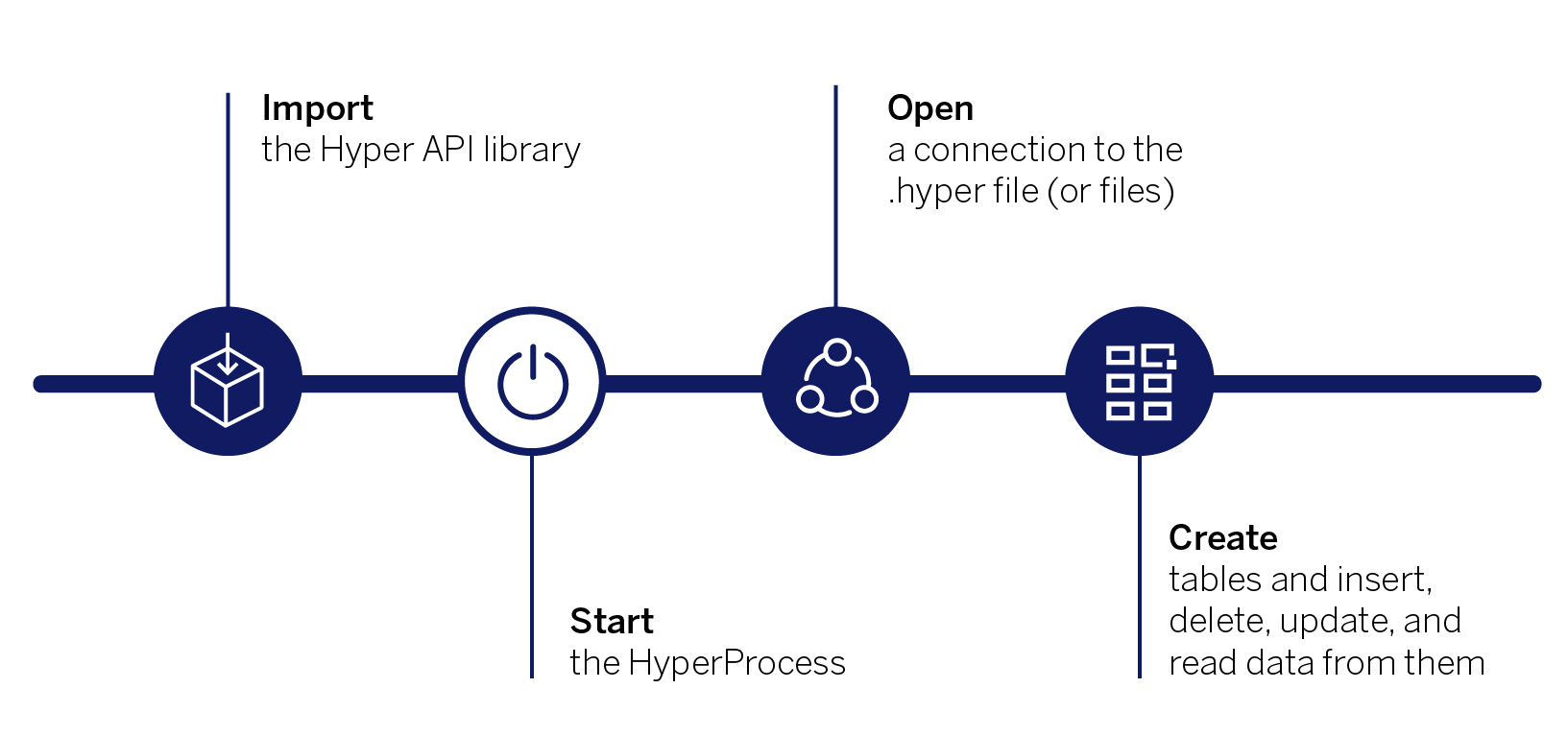
After you’ve imported or included the Hyper API library, you can start writing your code. The first step is to start up a local Hyper database server (hyperd
). Only one Hyper server instance should be running at any time. And as starting up and shutting down the server takes time, you should keep the process running and only close or shutdown the HyperProcess
when your application is finished. If you call the HyperProcess
in a with
statement (Python), using
statement (C#), scope
(C++), or try-with-resources
statement (Java), the hyperd
process will safely shutdown. While the HyperProcess
is running, however, you can create and connect to as many .hyper
files as you want.
Python example:
with HyperProcess(telemetry=Telemetry.SEND_USAGE_DATA_TO_TABLEAU) as hyper:
The HyperProcess
can be instructed to send telemetry on Hyper API usage to Tableau. To send usage data, set telemetry
to Telemetry.SEND_USAGE_DATA_TO_TABLEAU
when you start the process. To opt out, set telemetry
to Telemetry.DO_NOT_SEND_USAGE_DATA_TO_TABLEAU
. See About Usage Data for more information.
About Usage Data
To help us improve Tableau, you can share usage data with us by setting telemetry
to Telemetry.SEND_USAGE_DATA_TO_TABLEAU
when you start the process. Tableau collects data that helps us learn how our products are being used so we can improve existing features and develop new ones. All usage data is collected and handled according to the Tableau Privacy Policy.

You use a connection object to connect to a .hyper file (also known as database file) by providing the (relative) path to the file. The connection object is also used to create new .hyper files and immediately connect to them. You can create as many connection
objects and can connect to as many .hyper
files as you need to, provided that there is only one connection per .hyper
file. You can also set other options, for example, to overwrite the file if it exists. The Connection
constructor requires a HyperProcess
instance to connect to. If your application creates multiple connections, each connection should use the same HyperProcess instance.
Again, if you create the connection using a with
statement (in Python), when the with
statement ends, the connection closes. The with
construct means we don’t have to call connection.close()
explicitly. You should always close the connection when your application is finished interacting with the .hyper
file.
Python example:
with Connection(hyper.endpoint, 'MyExample.hyper', CreateMode.CREATE_AND_REPLACE) as connection:
In this sample code, the CreateMode
specifies that we want to do if the .hyper
file already exists. In this case, we want to create the file (MyExample.hyper
) if it doesn’t exist and replace it (overwrite it) if it does (CreateMode.CREATE_AND_REPLACE
). If you just want to update or modify an existing file, you would choose CreateMode.NONE
. CreateMode.NONE is used to read data from an .hyper file.

Create the table definition
Create the table definition(s) using the TableDefinition
class and name the table.
Python example:
connection.catalog.create_schema('Extract') example_table = TableDefinition( TableName('Extract','Extract'), [ TableDefinition.Column('rowID', SqlType.big_int()), TableDefinition.Column('value', SqlType.big_int()), ])
You can find more information on how to create schema here.
Create the table(s)
You create a table using the connection's catalog. The catalog is responsible for the metadata about the extract (database) file. You can use the catalog to query the database.
Python example:
connection.catalog.create_table(example_table)
This sample code creates the table we defined in the previous step.
Add data to the table(s)
You can populate the table using the Inserter class
, or use SQL commands to copy or add data.
Python example:
with Inserter(connection, example_table) as inserter: for i in range (1, 101): inserter.add_row( [ i, i ] ) inserter.execute()
The inserter.execute()
method has to be called to actually insert all the rows in to the .hyper file. The execute() method should be called once at the end of the insertion process of all rows and not for every individual row.
Read data from the hyper file
Using the Hyper API, you can read data from tables in an .hyper
file by sending SQL queries. Indeed, the Connection
class in the Hyper API provides methods for executing SQL statements and queries. The Hyper API provides three methods that are specific to queries.
Python example:
The syntax for these SQL query methods is as follows
connection
.execute_query(query="sql_query_string"
)
To learn more about how to read data from Hyper Files, read our documentation here.
Insert, Update, and Delete Data
Using the Hyper API, you can insert, update, and delete data from tables in an .hyper
file by sending SQL commands and queries, and by using the Inserter
class to add rows to a table.
To learn more about the Inserter class and Hyper SQL commands for inserting, updating, and deleting data from a Hyper file here.
Close the connection and shutdown the HyperProcess
When your application is finished populating the extract file with data, you first close the connection you opened to the database (the .hyper
file) and shutdown the HyperProcess
.
Related Resources
- Tableau Hyper API
- Tableau Hyper API Reference
- Code Samples (GitHub)
- Use SQL Commands with Hyper Files
- Insert Data Directly from CSV Files
- Hyper API: Automating Data Connectivity to Solve Real Business Problems
- Turbo-charged Extract Creation & Modification: A Technical Introduction into the Hyper API
- Pantab: A Python wrapper around Tableau’s Hyper API which promotes usage of the pandas DataFrame to seamlessly generate and read hyper extracts.
上次更新: 7月31日